Back to all functions
Send a SMS message via Twilio
This function sends an SMS message to a specified phone number using Twilio's messaging service.
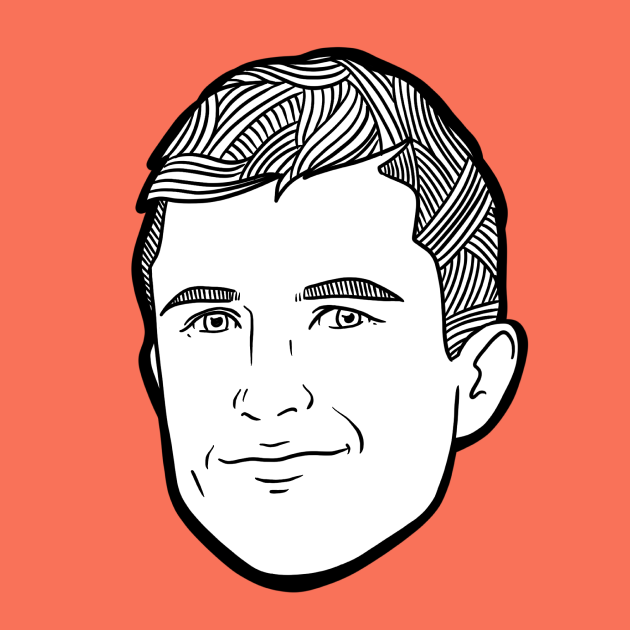
.webp)
Created By
Zoran Slamkov
Voiceflow
INPUT VARIABLES
{
twilioAuthtoken
}
{
twilioAccountSid
}
{
messageBody
}
{
}
{
}
{
}
OUTPUT VARIABLES
{
}
{
}
{
}
{
}
{
}
{
}
PATHS
{
fail
}
If something goes wrong running the function, it will use this path
{
success
}
If the query was made successfully, this path is chosen.
{
}
{
}
{
}
{
}
Function Code Snippet
export default async function main(args) {
// Extract necessary variables from args
const { phoneNumber, messageBody, twilioAccountSid, twilioAuthToken, twilioNumber } = args.inputVars;
// Custom Base64 encoding
function base64Encode(str) {
// Define the character set for Base64 encoding
const charSet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/';
let base64 = '';
for (let i = 0; i < str.length; i += 3) {
const byte1 = str.charCodeAt(i);
const byte2 = str.charCodeAt(i + 1) || 0;
const byte3 = str.charCodeAt(i + 2) || 0;
const enc1 = byte1 >> 2;
const enc2 = ((byte1 & 0x03) << 4) | (byte2 >> 4);
const enc3 = i + 1 < str.length ? ((byte2 & 0x0f) << 2) | (byte3 >> 6) : 64;
const enc4 = i + 2 < str.length ? (byte3 & 0x3f) : 64;
base64 += charSet.charAt(enc1) + charSet.charAt(enc2) + charSet.charAt(enc3) + charSet.charAt(enc4);
}
return base64;
}
// Construct the POST data string for the Twilio API request
const postData = `To=${encodeURIComponent(phoneNumber)}&From=${encodeURIComponent(twilioNumber)}&Body=${encodeURIComponent(messageBody)}`;
// Twilio API endpoint for sending SMS
const twilioUrl = `https://api.twilio.com/2010-04-01/Accounts/${twilioAccountSid}/Messages.json`;
// Prepare the authorization header using the custom base64 encoding function
const encodedCredentials = base64Encode(`${twilioAccountSid}:${twilioAuthToken}`);
// Set up the headers for the Twilio API request
const headers = {
'Authorization': `Basic ${encodedCredentials}`,
'Content-Type': 'application/x-www-form-urlencoded'
};
// Send the SMS message using Twilio's API
try {
const response = await fetch(twilioUrl, {
method: 'POST',
headers: headers,
body: postData
});
// Access the response directly (Voiceflow's modification to the standard Fetch API)
const jsonResponse = response.json; // Accessing the JSON response
// Handle the Twilio response here
if (jsonResponse && jsonResponse.sid) {
// Message sent successfully
return {
next: {
path: 'success'
},
trace: [{
type: 'text',
payload: {
message: `Message sent successfully to ${phoneNumber}`
}
}]
};
} else {
// Message failed to send
return {
next: {
path: 'fail'
},
trace: [{
type: 'text',
payload: {
message: `Failed to send message: ${jsonResponse.message}`
}
}]
};
}
} catch (error) {
// Handle fetch errors
return {
next: {
path: 'fail'
},
trace: [{
type: 'text',
payload: {
message: `Error sending message: ${error.message}`
}
}]
};
}
}
Function Walkthrough
Explore More Functions
Build and submit a Function to have it featured in the community.
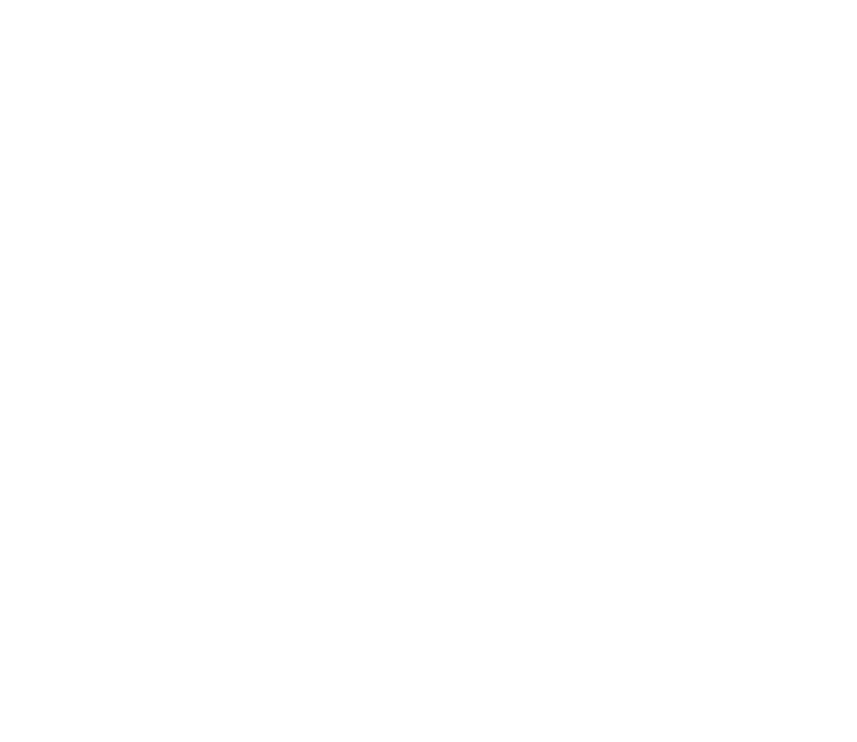